06 May 2021
Practical code examples of why Typescript is the best choice for frontend
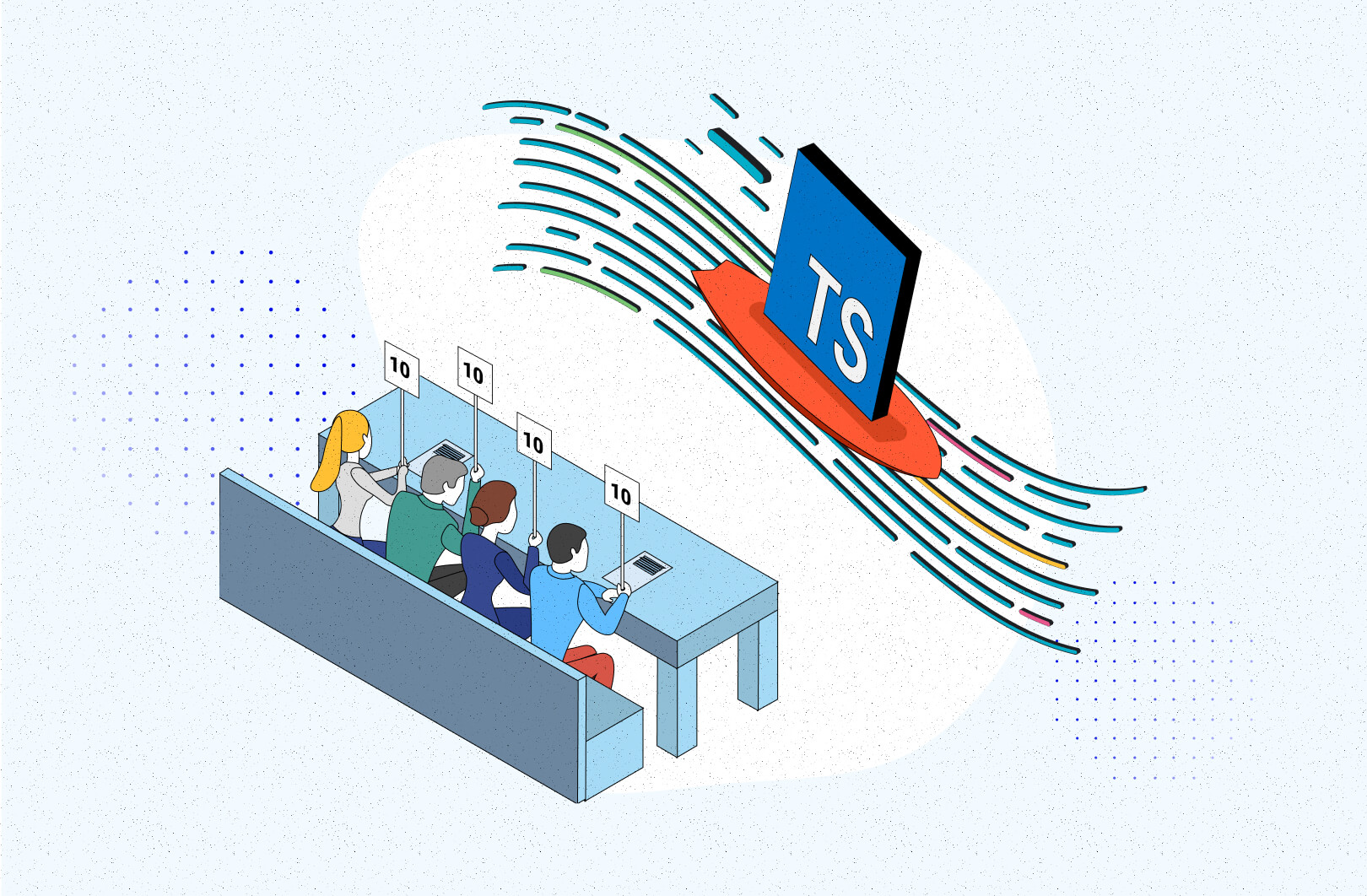
In my previous article: “Why use TypeScript” I discussed why Typescript code is the best for frontend in 2023 in a very understandable way, even to non-technical people. If you weren’t happy with the lack of code in my previous article, buckle up, because we’re up for a ride! Now it’s time to take a look at some practical examples.
React + MUI + Typescript = <3
In this blog post, I will go through some code snippets based on React, which is the most popular and widely used frontend library at this moment. Sources? Check out our analysis of two reports:
- JavaScript trends in 2023 according to “State of JS”,
- Frontend technologies in 2021 based on “State of Frontend”.
Additionally, I used here MaterialUI library for React. I know I’m discussing only React code examples here, but I believe that this is additional proof that Typescript is the best choice even for other frameworks. Think Vue (especially Vue3) or Angular.
Without further ado, let’s go to the first example of Typescript makes code easier.
Example no. 1: Generic reusable list with render props
Lists are one of the most common things that exist in frontend applications. You can see them everywhere online, e.g. list of posts on Facebook, list of files in Google Drive, list of articles on our TSH blog… 🙂
If you’re building some bigger frontend application, you will need to render the same looking list in different places that differ only by the content inside the list item. You have to build a frontend for the university library with a reusable component for the grid list. The component needs to be the only place with styles definition, and when you’ll have to change anything in future, this will apply to all of your lists across the whole application. Let’s prepare some students and books example data.
Students’ example data:
Books example data:
Notice that these two types differ from each other (and probably there will be other data types when implementing other lists, e.g. authors, categories). You could create two separate components for each list that loops through all items, and it would probably work fine. But let’s try to use Typescript generics and React render props pattern to achieve one component reusable across the whole project where we can pass lists of any items and only define how a single grid tile should look like.
Check out the code below:
OK, so what happened here? Let’s investigate this example step by step.
First, define BaseListItem
type which contains id only
This is the type that I used to define all required properties which should exist in every item passed to our list. Unique id for every database record is just standard so for sure we will have it here. This id property will also serve us to be the key of each list item.
The next thing is the GridListProps
type for your grid list component props
Now things are getting more interesting. 🤔 Here you can see that I used Typescript generics functionality. It may seem scary for someone who hasn’t worked with generics before but let me explain what happens here.
This is the generic type where I defined that whatever type will be passed here, it must contain id property because it extends my previously created BaseListItem
type. This type contains two properties: items
– an array of whatever type is passed to this generic, and renderItem
– a function that returns ReactNode
(so probably some other component or JSX).
Pro tip about TypeScript generics
Use descriptive names for Typescript generics. If you worked with generics, you’ve probably seen that many libraries use generic names like T, P, R, U etc. Of course, it’s shorter than any meaningful name, but trust me, your colleagues will thank you for naming convention understandably, even at first glance.
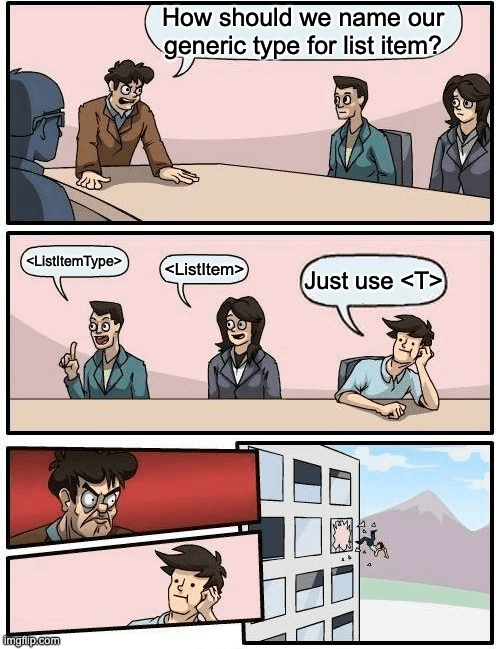
Lastly, define generic functional component GridList
As you can see here, I destructured items
and renderItem
props. This component loops through all items and calls renderItem
function with the item
as an argument.
Now with component prepared like this, you can reuse it with your previous prepared mock data like this:
Students List Component
Books List Component
And in your app, you can render these lists like this:
You can reuse the same component for any list you can imagine. And achieve the following benefits:
- The same look of every list built with this component. You only define how the content of the grid item should look like,
- Full Typescript code autocompletion support for any kind of objects,
- Assurance that every list item has an id property which is also key for this list item which is important for list elements identity in React.
Pro tip:
In StudentsList and BooksList components I explicitly provided type. Remember that you don’t have to do this because Typescript can infer the generic type based on items you passed. As seen in the gif below.
💡 More Typescript? No problem, check this out:
Why Typescript is the best frontend language?
Summarizing our quick yet mysterious journey across React with the Typescript world you can see with just these two simple examples that Typescript gives you really powerful tools to use in your daily work. With Typescript, you gain full control over creating what you want from A to Z.
We’ve gone through a bunch of Typescript features like creating custom types, generics, union types, intersections, types inference but there are many more things to discover. But I bet you wouldn’t stay here for another hour. 😀
Nevertheless, if you are interested in Typescript I encourage you to check out its official documentation. Inside you’ll find more examples and use cases of all features that will help you build the app of your dreams. I hope now you are fully convinced that Typescript is the best choice to write frontend applications. Especially with React. 💙
Trying to stay up-to-date? TechKeeper's Guide will save you a lot of time
We know how much effort it takes not to fall behind with new technologies. Our newsletter means hand-picked and condensed news in your inbox, once every two weeks. You will never miss anything big from the IT world for sure. 📰