07 June 2023
Node.js interview questions, topics and advice – nail your interview in 2023
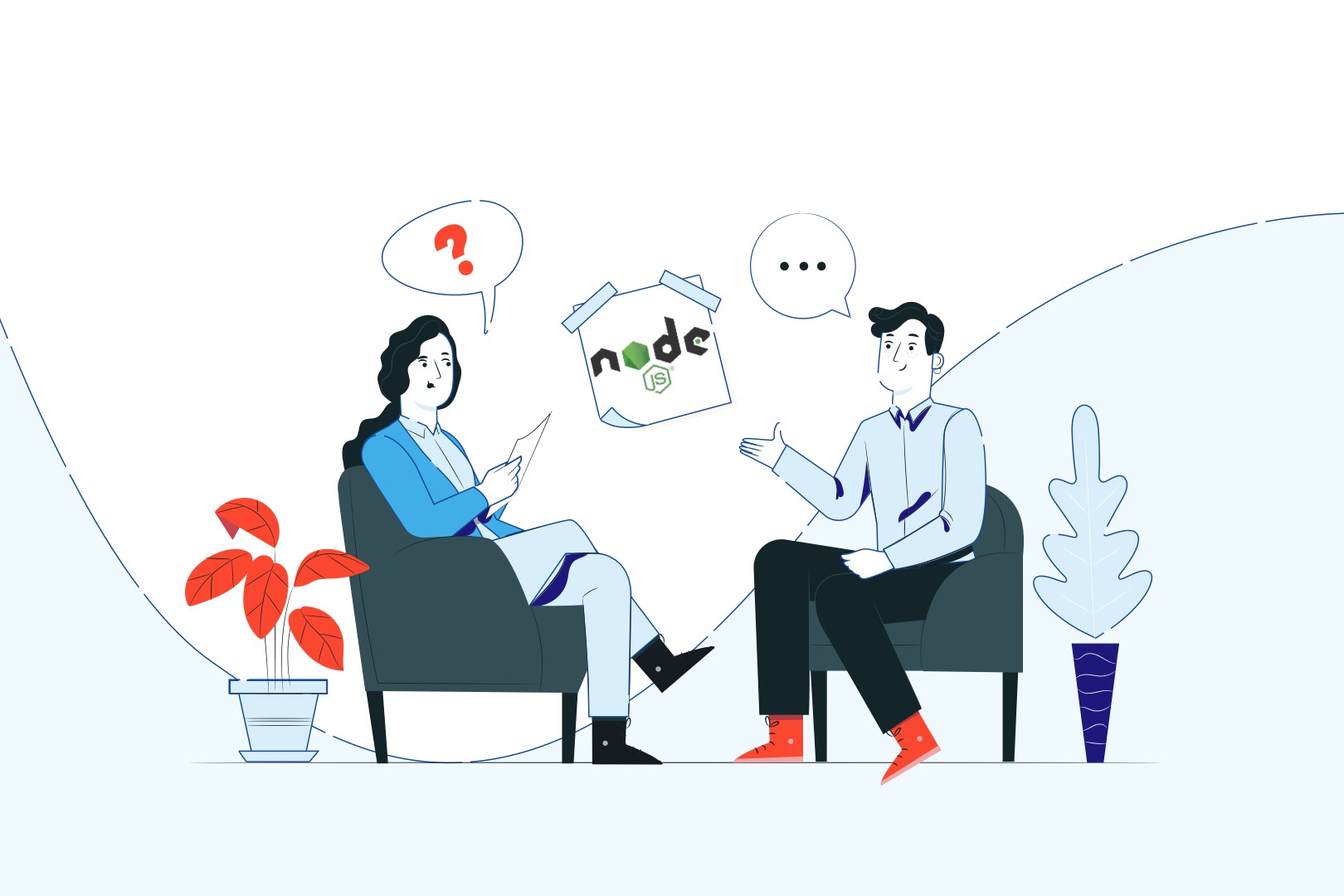
Node.js has been one of the most consistently in-demand technologies for the past couple of years. But since many people who are learning it come from both the backend and frontend, it doesn’t mean that it is easy to get the dream Node.js job. That’s why you need to prepare yourself well if you want to nail Node.js interview questions. In this article, you will find some questions… and reasons as to why the questions themselves aren’t the most important aspect.
As an experienced Node.js developer and a current VP of Technology at The Software House, a software company that made Node.js one of its most important specializations, I’m always looking for interesting prospects.
The process which I helped develop is meant to find Node.js developers with the best long-term potential. The Node.js interview is a big part of that.
Below, I’m going to show you some areas of expertise that are currently of the most importance for Node.js developers. There will be some Node interview questions too, but keep in mind that memorizing Node.js questions and answers alone won’t get you far. Firstly, you can’t predict the exact questions you’ll be asked. What’s more, the gaps in your knowledge will eventually surface and then, there are also soft skills that require practicing in their own rights. Focus on improving your general ability and experience in each of these areas.
That’s why in this article, I’m going to show which areas we focus on and why. Naturally, there will be examples of Node interview questions and answers for each of these areas as well. The complete list of Node.js areas of interest include:
- JavaScript and TypeScript – at TSH, we absolutely love working with TypeScript – static typing makes a big difference, but there’s more to that,
- Node.js-related frameworks (e.g. Express) – the Node.js environment of tools and techniques continues to expand and become ever more useful,
- REST/GraphQL/WebSockets – APIs today are of great importance so make sure to brush up on that,
- Architecture-related questions – this one is a no-brainer,
- Database questions – both relational and non-relational databases are essential to know,
- Testing – with all the complexity that microservices bring, no wonder that testing microservices is such a big deal,
- Design patterns – the practical knowledge of design patterns is the shortest route to actually mastering microservices,
- DevOps questions – our DevOps team is well-versed when it comes to microservices-based infrastructure. As an AWS Advanced Partner, we often work with microservices on the AWS cloud.
- Soft skills – working with other people efficiently, sharing knowledge, speaking English – all of those skills are put to everyday use at The Software House.
All right then – let’s get right to it!
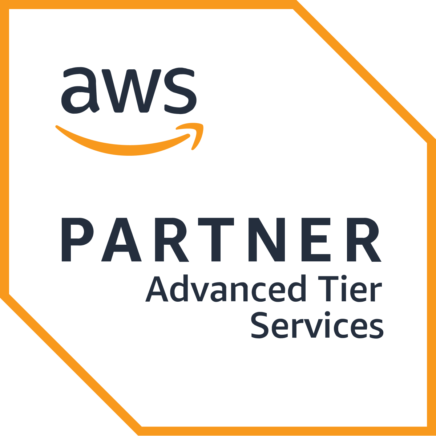
JavaScript/TypeScript questions
If you want to develop in Node.js, a strong knowledge of JavaScript is an absolute must. The fundamentals are what make the most difference. They include types of scopes, closures, and differences between various types of variable declarations as well as creating all kinds of functions. All of this has a great impact on the quality of the code you write.
You should also learn more about TypeScript if you haven’t yet. Nowadays, developers write more and more Node.js applications in this language. It’s true for the devs at The Software House as well.
And what are some of the typical TypeScript questions or tasks you can get during a Node.js interview?
- Why and when would you use TypeScript in place of JavaScript?
- Does TypeScript support all principles of object-oriented programming?
- Explain why a particular piece of code is marked wrong by the code validator (make sure to practice a lot of examples of these).
Node.js/Frameworks questions
It’s vital to understand all the basics of Node.js itself – how the event loop works, why you should avoid synchronous methods and more. Working with Node.js is a lot about handling APIs and the framework you should be familiar with is express.js. That’s why you should definitely brush up on error handling in express.js and the mechanics of the middleware.
What are some typical questions?
- How can you improve the scalability of a Node.js app?
- How does the Express middleware work?
- When should you use asynchronous and synchronous methods?
- What is Node.js callback function? What are asynchronous function calls used for? Can you mention some practical use cases for callback functions in Node.js?
- What is the event loop for in Node.js?
- Can you explain how event driven programming on Node.js works?
- What arguments does async.queue take as as input (task function concurrency value)?
- What is the Buffer class and why should you explicitly reference it via an import or a require statement?
- What is async processing in Node.js?
- What is a single threaded event loop used for?
- What are the uses of the control flow function in Node.js?
- What are the best Google Chrome DevTools for debugging Node.js applications?
- What do you know about the mechanics of Node.js code execution? What do you know about Node.js’s built-in HTTP module?
- What is the Node.js file system module?
REST/GraphQL/WebSockets questions
Most Node.js applications are various types of APIs. Therefore, your knowledge in this area will be crucial. Until recently, the experience with REST API, including authentication methods and mapping of HTTP methods onto endpoints, was sufficient. Nowadays, you also need to know a lot about GraphQL and WebSocket. Expect to be asked about which approach might be the best in a given situation. Scalability and optimizing the number of operations can also show up during the Node.js interview.
How about some examples of Node.js interview questions?
- Explain the key concepts of GraphQL such as product-centric, hierarchical or client-specified queries.
- Describe the most popular authorization methods for APIs.
- How would you decide which API technology to choose for a given project?
Architecture questions
Each and every app has some kind of architecture that has an impact on how it works and how to best proceed with the development. It’s a good idea to learn about various types of monolithic apps and especially about microservices. Some typical areas may include: methods of communication used by the application is it direct only or more, does it use events/queues, etc.
Now, some questions:
- What kind of performance gains can you achieve by switching from a monolithic architecture to microservices? Where do they come from?
- What was the most complex architecture you worked with? Can you describe it?
- How does CQRS work and when is it worth it to implement it?
Do you need even more unique microservices insights?
Our State of Microservices 2020 report shows just how well Node.js goes with this type of architecture. Check out a work created with the help of over 650 tech leaders!
Database questions
You’d be hard-pressed to find an app that doesn’t store some kind of data. That’s why most likely you will be asked about what kind of databases you’ve worked with. Was your experience limited to relational databases (PostgreSQL, MySQL, etc.) or perhaps some NoSQL databases too (MongoDB, Elasticsearch)? Methods of query optimization may also likely show up during a Node.js interview.
Some typical Node.js database interview questions may be as follows:
- Describe the difference between optimistic locking and pessimistic locking.
- What are the main factors to consider when choosing a database for a project?
- The pros and cons of using non-relational databases.
Testing questions
There is no (good) application without testing. That’s why some level of knowledge about unit testing, integration testing as well as end-to-end testing is essential. Some of the Node.js interview topics related to testing may include mocking, injections, or the Test Pyramid.
Now, some interview questions related to testing:
- At which point in the development process should testing be implemented?
- What does the choice of your testing depend on?
- What are some specific challenges of implementing testing in Continuous Integration?
Design patterns questions
These days, more and more companies desire Node.js developers who understand the importance of design patterns. In many cases, just using the right design pattern can change poorly written and unscalable code into an easy-to-maintain and scalable module. At The Software House, we often ask about dependency injections as this is something we use a lot in our Node.js projects.
How about some design patterns-related Node.js interview questions?
- What are the pros and cons of using dependency injection?
- What is the difference between the observer pattern and the pub/sub pattern?
- How does the API Gateway pattern work? When is it a good idea to go for it?
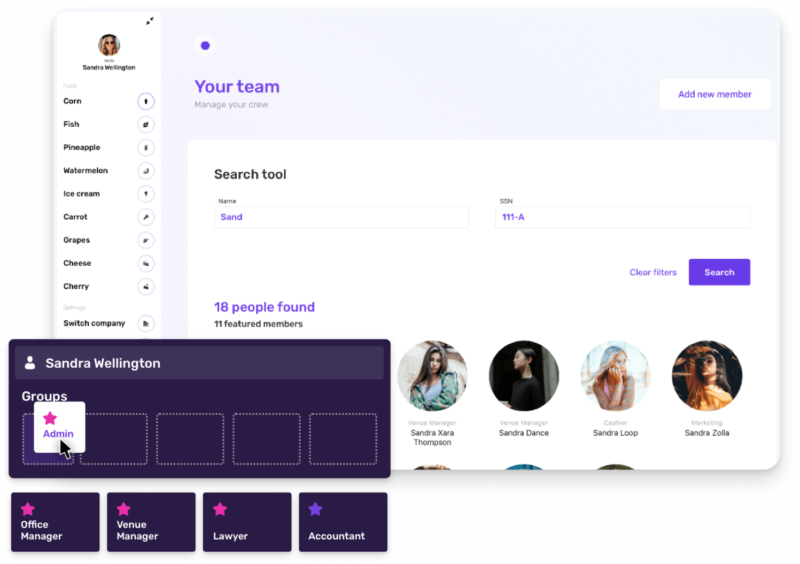
DevOps questions
A good Node.js developer can not only code, but also prepare a fully working development environment ready for Continuous Integration. If this is something important for the company you’re applying for, expect questions about app containerization and tools dedicated to automating CI processes.
DevOps-related Node.js questions may be something like:
- What kind of CI tools have you worked with?
- What is your experience with Docker?
- Do you have experience with AWS/GCP?
Soft skills questions
There are a lot of programmers, including technically skilled ones, who don’t pay much attention to soft skills. But these are in fact the skills that set apart a true senior dev from good mid developers. Hardly any developer works alone today. Most exist as a part of teams and being able to communicate well in such an environment makes a big difference.
You might expect questions like these during your Node.js interview:
- Have you ever worked as a mentor for a junior developer?
- Did you have direct contact with the client?
- How good is your English? 🙂
Node.js interview questions – conclusions
What do you think about these Node js areas? Besides them, you might also expect some JS interview questions. After all, Node.js is a JS-based software and your general proficiency in JavaScript code will be relevant. You might get a question about a popular JavaScript library such as jQuery, React, Vue.js and so on. You might get to see a vanilla JS file during practical exercises if they are included in the recruitment process.
Keep in mind that depending on the nature of the company/position you’re applying for, some of the areas can be more or less important. Try to estimate yourself which ones are the most crucial for the position you’re going for.
Learn more about the kind of Node.js development we’re doing at TSH. And if you are going for something else than Node.js, here you can find out more about the interview questions for PHP developers, frontend developers, as well as microservices-heavy positions.
Preparing for a job interview for a different position? Help incoming!
Are you also looking for a technological partner that could help you with your next Node.js project?
The Software House’s Node.js team is one of the biggest and most accomplished in Poland!